Overview
contactHeRo is a desktop contact management application made for Human Resource (HR) recruiters in a company. It contains several useful functions for the user like a Login system, Job listings system, Calendar and appointment system, and an Email system.
This portfolio is here to showcase my development of software engineering skills throughout the course of CS2103T as well as how I have applied these skills and knowledge into developing a real product with real users.
Summary of contributions
-
Major enhancement: added the ability to send out email in contactHeRo, using the Google API. Also provided a framework to integrate Google API.
-
What it does: allows the user to send email to their contact, using an in-app editor. To use the Google API to send email, users will have to login to Google. Our app provides a built-in browser to handle the user login and a framework to integrate Google API.
-
Justification: As we all know, recruiters will usually send out emails to potential employees to inform them of their job application progress or arranging for interviews. This feature lets user take advantage of the fact that the information of the potential employees are already in the app, therefore it will be easier to draft an email than using other email applications. It also provides a better user experience as users do not need to switch to another application.
-
Highlights: This enhancement provides a framework for future feature which requires Google API, like Google Contacts, Google Drive or Google Calendar. It required the programming ability to integrate third-party API and third-party code into our own app. The implementation was challenging as you do not have control over the source code in the third-party API, which means you have to work your own code around it.
-
Credits: stackoverflow.com, googledevelopers.com
-
-
Minor enhancement: added the ability to find by tag, which allows the user to find their contact using the skills tag.
-
What it does: It allows the user to search for a contact based on their skills tag.
-
Justification: Sometimes the user might not remember the name of the person they have shortlisted. The skills required for the job is easier to remember and therefore, this feature is very useful in helping them find a contact easily.
-
Highlights: This enhancement allows me to familiarise myself with the code base of Addressbook Level 4, as the Find command touches on multiple components of the app. It required the ability to read and understand codes written by others and also adding your own contributions to the codebase.
-
-
Code contributed: [Functional code] [Test code]
-
Other contributions:
-
Project management:
-
Reach all the milestones
v1.1
-v1.5
on GitHub. -
Created issues and milestones for the team.
-
Help to merge and review team member’s PR.
-
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
Tools:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Login to Google : googlelogin
You will have to login to google in order to use features like
emailing. This process is simple and fast like how you would normally login
using the web browser.
Format: googlelogin
Examples:
-
googlelogin
This will open up theGoogle
tab for you to login. On running the above command, you should see the following success message:Please log in to Google.
You should also see the login screen as shown below.
Please do not go to any other webpages in the Google tab after you have logged in.
|
Now that you have logged in, you are ready to use the Email
feature to send out emails!
Emailing your contact : email
You can send email to any person you have saved in contactHeRo using the following format.
Format: email INDEX [sub/EMAIL_SUBJECT]
Examples:
-
list
email 2
This will open up the Draft Email tab and then collect the information of the 2nd person in the list. -
list
email 2 sub/Interview on 13 May 2018
This will open up the Draft Email tab and then collect the information of the 2nd person in the list. It will also set the email subject title to "Interview on 13 May 2018".
On running the above commands, you should see similar message like the following:
Drafting email to: berniceyu@example.com
The collected information will be used automatically to fill up details as shown below in figure 5.
If you have also used sub/Interview on 13 May 2018
in the command, the Subject textbox will also be fill with "Interview on 13 May 2018".
You can use keyboard short-cuts like Ctrl-B to bold your text while drafting
your email.
|
Finally, after you are done drafting up the email, simply hit the Send
button to send your email. If the email is sent successfully, you should see
a pop-up message as shown below.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Emailing a contact using Google API
Current implementation
The sending of email will be implemented using the Google API.
Firstly, to access the Google API, the user must login to Google using the googlelogin
command.
The GoogleLoginCommand
class will open the Google authentication webpage in the built-in web browser for user to login.
The GoogleAuthentication
class will handle the authentication process of Google login.
After authentication is successful, the user will be redirected to the Google homepage,
where the Token
is stored in the URL.
Below is the sequence diagram of the googlelogin
command:
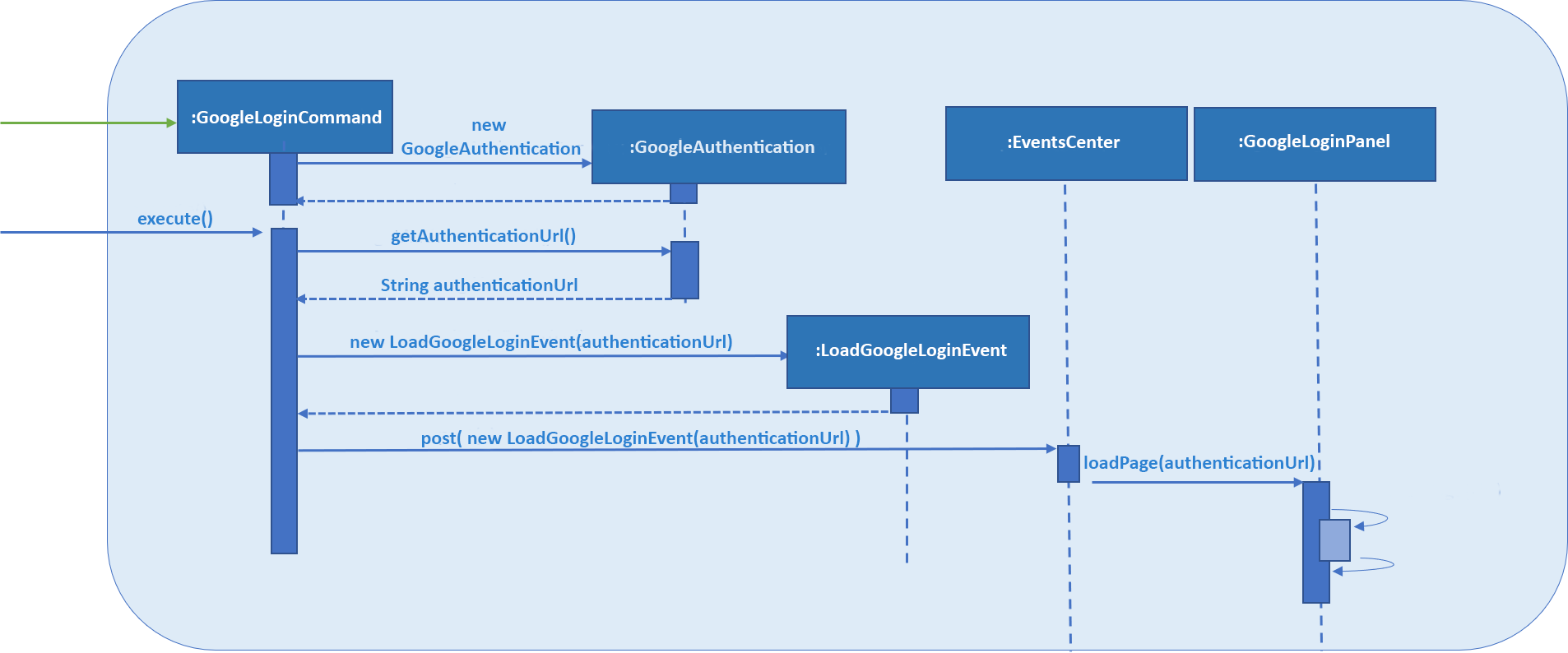
Next, a GmailClient
is created using the GoogleAuthentication
class and Token
.
The Token
is obtained from the redirected URL, meaning that the user must not navigate to other URL
after they have logged in.
Below is the class diagram of the GmailClient
:
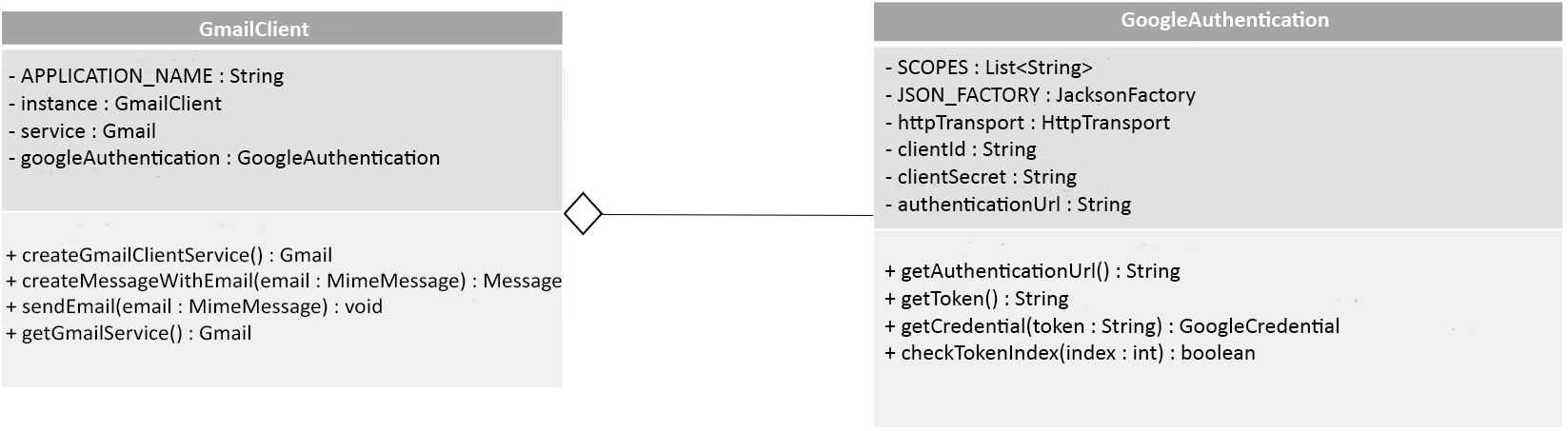
This newly created GmailClient
class will provide all the services that use the Google API.
Now that the GmailClient
is created, we can use it send out emails!
The user will use the email
command to start this process.
The EmailCommand
class will open an email interface to send email to the chosen contact. EmailCommand
extends the Command
class. It takes in an index and also an optional field EMAILSUBJECT
through the EmailCommandParser
,
similar to the implementation of EditCommand
and EditCommandParser
.
The EmailPanel
is the UI for user to draft their email. The UI has 3 inputs, To, Subject and Body. From is not needed here as we already have
the authorised user’s email address in GoogleAuthentication
.
The GmailMessage
class will take in the values input by the user
in the UI. These values will be stored in GmailMessage
and packaged into a MimeMessage
ready to be send out.
public static MimeMessage createEmailContent(String to, String from, String subject, String bodyText) throws MessagingException {
Properties props = new Properties();
Session session = Session.getDefaultInstance(props, null);
MimeMessage emailContent = new MimeMessage(session);
emailContent.setFrom(new InternetAddress(from));
emailContent.addRecipient(javax.mail.Message.RecipientType.TO, new InternetAddress(to));
emailContent.setSubject(subject);
emailContent.setContent(bodyText, "text/html; charset=utf-8");
return emailContent;
}
Finally, GmailClient
will send out the MimeMessage
of GmailMessage
as an email.
public static void sendEmail(MimeMessage emailContent) throws MessagingException, IOException {
Message message = createMessageWithEmail(emailContent);
message = service.users().messages().send("me", message).execute();
}
Design Considerations
Aspect: How to login to Google
-
Alternative 1: Using
GoogleAuthorizationCodeFlow
to log in.-
Pros: Very simple to implement and handle.
Token
andCredential
are handled by the API. -
Cons: It opens a default web browser, which the users are able to close.
GoogleAuthorizationCodeFlow
will stall the main thread in order to wait for the user to authenticate, if the user close the browser, the app will crash.
-
-
Alternative 2 (current choice): Using a built-in web browser to get the
Token
from the URL.-
Pros: User cannot close the built in browser. The authentication process will not stall the main thread.
-
Cons: If the user changes the URL in the web browser, the
Token
is gone and the user have to re-login.
-
Aspect: How to send an email
-
Alternative 1 (current choice): Using Gmail API to send an email.
-
Pros: Able to customize the UI that the user will use to send out an email.
-
Cons: Will take more time to implement due to additional UI components.
-
-
Alternative 2: Using
Webview
to display the Gmail drafting url.-
Pros: Faster implementation as similar feature has been done before.
-
Cons: Not customizable and text may appear small in
Webview
.
-
Enhancement Proposed (for Version 2.0)
-
Ability to add attachments for email.
-
Allow the user to set email content using the appointment in calendar.
Other Projects
Neighber - An Android app that allows users to borrow items from their neighbours. (Runner-up for NUS Orbital 2016/2017 - Apollo 11)